Samsung In-App Buy (IAP) provides builders a strong answer for dealing with digital transactions inside cellular functions accessible on Galaxy Retailer. Whether or not it’s promoting digital items, dealing with subscriptions, or managing refunds, Samsung IAP is designed to supply a clean, safe expertise. The Samsung IAP Orders API expands the scope of those advantages. You’ll be able to fetch all of the funds and refunds historical past based on specified dates. This content material guides you thru the important elements for implementing each the Samsung IAP and Samsung IAP Orders APIs.
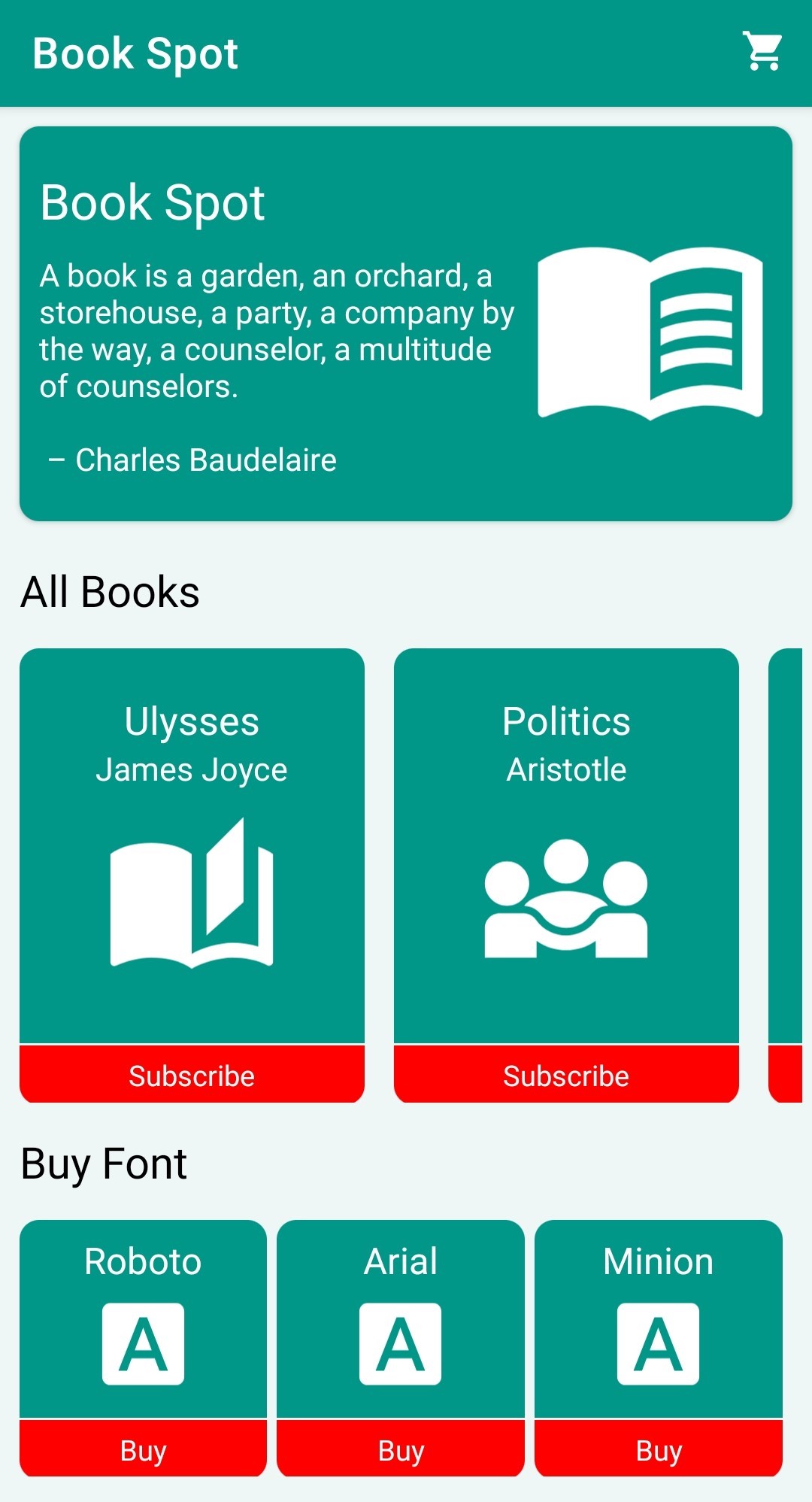
Determine 1: Pattern Software UI
On this tutorial, we offer a pattern software referred to as E-book Spot, which provides customers the choice to subscribe to their favourite books and consumable gadgets, akin to textual content fonts, for buy. After buy, customers can devour the merchandise. Lastly, builders can view all their cost and refund historical past on particular dates by calling the Samsung IAP Orders API from the back-end server.
Conditions
Earlier than implementing in-app purchases in your app, do the next to allow a clean and efficient execution of the method whereas growing your personal software:
- Combine the Samsung IAP SDK into your software. For extra details about the IAP SDK integration, you possibly can comply with the Integration of Samsung IAP Companies in Android Apps article.
- Add the applying for Beta testing on Samsung Galaxy Retailer. A step-by-step information with screenshots has been supplied within the documentation. For extra particulars, see the part “Manufacturing Closed Beta Take a look at” on the Take a look at Information.
- Lastly, create gadgets on the Vendor Portal in order that customers should buy or subscribe to them whereas utilizing the applying. For extra particulars in regards to the accessible gadgets that the Vendor Portal helps, see the Programming Information.
For the pattern software, we’ve already accomplished these steps. Some instance gadgets have been already created in Vendor Portal, akin to books and fonts so as to devour and subscribe to them whereas utilizing this pattern software.
Implementation of Merchandise Buy
Now that the applying and gadgets are prepared, you possibly can implement the acquisition performance in your software like within the pattern beneath:
- When clicking “Purchase,” the
startPayment()
technique is named, specifying parameters for merchandise ID and theOnPaymentListener
interface, which handles the outcomes of the cost transaction. - The
onPayment()
callback returns whether or not the acquisition has succeeded or failed. ThepurchaseVo
object is instantiated and in case it isn’t null, it holds the acquisition outcomes. - If the acquisition is profitable, then it validates the acquisition exhibiting its ID. If the acquisition will not be profitable, a purchaseError message is proven. For extra data, examine the Buy an in-app merchandise part.
iapHelper.startPayment(itemId, String.valueOf(1), new OnPaymentListener() {
@Override
public void onPayment(@NonNull ErrorVo errorVo, @Nullable PurchaseVo purchaseVo) {
if (purchaseVo != null) {
// Buy Profitable
Log.d("purchaseId" , purchaseVo.getPurchaseId().toString());
Toast.makeText(getApplicationContext() ,"Buy Efficiently",
Toast.LENGTH_SHORT).present();
} else {
Log.d("purchaseError" , errorVo.toString());
Toast.makeText(getApplicationContext() ,"Buy Failed",
Toast.LENGTH_SHORT).present();
}
}
});
Implementation of Merchandise Consumption
After efficiently buying an merchandise, the person can then devour it. Within the pattern code beneath, when “Consumed” is chosen, the consumePurchaseItems()
triggers the devour performance. That is crucial as gadgets have to be marked as consumed to allow them to be bought once more:
- The
consumePurchaseItems()
technique is named specifying the parameters forpurchaseId
and theOnConsumePurchasedItemsListener()
interface, which handles the merchandise information and outcomes. - This code additionally checks if consuming the bought gadgets succeeded or failed:
- If the
errorVo
parameter will not be null and there’s no error with the acquisition, which could be verified with theIAP_ERROR_NONE
response code, then the “Buy Acknowledged” message is displayed. - Nonetheless, if there’s an error, the
errorVo
parameter returns an error description with thegetErrorString()
getter, together with the “Acknowledgment Failed” message.
- If the
iapHelper.consumePurchasedItems(purchaseId, new OnConsumePurchasedItemsListener() {
@Override
public void onConsumePurchasedItems(@NonNull ErrorVo errorVo, @NonNull
ArrayList<ConsumeVo>arrayList) {
if (errorVo != null && errorVo.getErrorCode() == iapHelper.IAP_ERROR_NONE) {
Toast.makeText(getApplicationContext() ,"Buy Acknowledged",
Toast.LENGTH_SHORT).present();
} else {
Toast.makeText(getApplicationContext(), "Acknowledgment Failed: " +
errorVo.getErrorString(), Toast.LENGTH_SHORT).present();
}
}
});
Implementation of Merchandise Subscription
In addition to buying and consuming gadgets, you can too subscribe to them in your functions. Much like the validation finished for the consumable merchandise buy, you validate the subscription with a purchase order ID if the acquisition is profitable. Use the identical code snippet specified for “Merchandise Buy.” For extra data, examine the Implementation of Merchandise Buy part.
Implementation of the Samsung IAP Orders API
The Samsung IAP Orders API is used to view all funds and refunds on a selected date. It does this by fetching the funds and refunds historical past throughout the date you specified. Let’s implement the Samsung IAP Orders API and create a server to take heed to its notifications. By way of server-to-server communication, the API returns all orders information for the applying.
Configuring the Server
You’ll be able to develop a Spring Boot server for this function. Listed below are the rules on learn how to arrange this server:
- Arrange a Spring Boot Challenge. For extra data, comply with the steps on Creating Your First Spring Boot Software.
- Arrange your server endpoint:
-
Create a controller for the Samsung IAP Orders API in an built-in growth setting (IDE) after importing the Spring Boot mission you created. This helps managing all in-app order-related actions and processing them inside your software.
-
The controller receives
POST
requests despatched from Samsung’s IAP orders service guaranteeing the communication together with your software.
-
Get Fee and Refund Historical past
To view all funds and refunds:
- You should make a
POST
request to the Samsung IAP Orders API endpoint with the required headers specified beneath. - If you happen to specify a date, all of the cost historical past for this date is returned. In any other case, it solely returns all the info from the day earlier than the present date.
API Endpoint: https://devapi.samsungapps.com/iap/vendor/orders
Methodology: POST
Headers:
Add the next fields to the request header. For extra data, see the Create an Entry Token web page, which helps you perceive learn how to create the entry token intimately. The token is used for authorization. It’s also possible to get the Service Account ID
by clicking the Help > API Service tabs on Vendor Portal. For extra particulars, learn the part Create a service account and go to Vendor Portal.
Header Title | Description | Required/Elective | Values |
---|---|---|---|
Content material-Sort | Format of the request physique | Required | software/json |
Authorization | Authorization safety header | Required | Bearer: access_token |
Service Account ID | This ID could be created in Vendor Portal and is used to generate the JSON Net Token (JWT) | Required | service-account-id |
Parameters:
The next parameters can be utilized to construct your POST
request.
Title | Sort | Required/Elective | Description |
---|---|---|---|
sellerSeq | String | Required | Your vendor deeplink, which is present in your profile in Vendor Portal and consists of a 12-digit quantity. |
packageName | String | Elective | Used to view cost and refund information. You’ll be able to present the applying package deal identify. When a package deal identify will not be specified, the info for all functions is proven. |
requestDate | String | Elective | Specify a date from which to view the cost and refund information. If the date will not be specified, the info from a day earlier than your present date is returned. |
continuationToken | String | Elective | Use this if you wish to examine if there’s a continuation for the info on the following web page. If there isn’t a extra information, the response is null. |
To implement REST API help, add the next OkHttp
library dependencies to your software’s construct.gradle
file:
implementation 'com.squareup.okhttp3:okhttp: model'
implementation 'com.google.code.gson:gson: model'
An in depth description of the request gadgets could be discovered within the Request part of the Samsung IAP Orders API documentation. For extra data on the server communication, see Samsung IAP Server API. Here’s a temporary abstract of the code beneath:
- A
POST
request is mapped to the/orders
URL, which logs the request. - The beforehand described parameters containing the info you specified are formatted in a JSON physique utilizing the
String.format()
technique. - The outgoing request is logged in a
JSON
physique format. - A
RequestBody
is instantiated containing theJSON information
, formatted for an HTTP request to be despatched to the server with the desired token and Service Account ID. - This code additionally handles a number of outcomes your request can return:
- The
onFailure()
technique is named when the community request fails for some cause, offering any error particulars utilizing theIOException
exception. - If the request succeeds, the
onResponse()
technique returns the response physique or any response exception discovered.
- The
@RestController
@RequestMapping(worth = "https://developer.samsung.com/iap", technique = RequestMethod.POST)
public class OrdersController {
personal remaining OkHttpClient shopper = new OkHttpClient();
@GetMapping("/orders")
public void sendToServer() {
System.out.println("POST request acquired"); // Log the request
// Outline parameters values, use based on your requirement
// String packageName = "com.instance.app_name ";
// String requestDate = "20240615";
// String continuationToken = "XXXXXXXXXXX…….XXXXXX";
String sellerSeq = "0000000XXXXX";
// Create the JSON physique, use packageName, requestDate, continuationToken based on your requirement
String jsonBody = String.format(
"{"sellerSeq":"%s"}",
sellerSeq
);
// Create the request physique
RequestBody physique = RequestBody.create(jsonBody, MediaType.parse("software/json; charset=utf-8"));
// Entry token
String token = "0DjT9yzrYUKDoGbVUlXXXXXX";
// Construct the request
Request request = new Request.Builder()
.url("https://devapi.samsungapps.com/iap/vendor/orders")
.put up(physique)
.addHeader("Authorization","Bearer " + token)
.addHeader("service-account-id", "85412253-21b2-4d84-8ff5-XXXXXXXXXXXX")
.addHeader("content-type", "software/json")
.construct();
shopper.newCall(request).enqueue(new Callback() {
@Override
public void onFailure(@NotNull Name name, @NotNull IOException e) {
System.err.println("Request failed: " + e.getMessage());
}
@Override
public void onResponse(@NotNull Name name, @NotNull Response response) throws IOException {
if (response.isSuccessful()) {
String responseBody = response.physique().string();
System.out.println("Response: " + responseBody);
} else {
System.err.println("Surprising response code: " + response.code());
System.err.println("Response physique: " + response.physique().string());
}
response.shut(); // Shut the response physique
}
});
}
}
Congratulations! You have got simply constructed the Spring Boot server to deal with API POST requests utilizing the OkHttpClient to handle HTTP requests and responses on your pattern software.
Instance Response
As beforehand talked about, a JSON-formatted response is returned to your request. For detailed descriptions of every response physique component, see the “Response” part of the Samsung IAP Orders API documentation. The next output format is a pattern wherein solely a few of the response-body information is offered.
- On this case, the
continuationToken
parameter key returns null as a result of there isn’t a continuation for the info on the following web page. - The
orderItemList
parameter key lists all of the orders with particular particulars, akin toorderId
,countryId
,packageName
, amongst others.
{
"continuationToken": null,
"orderItemList": [
{
"orderId": "S20230210KR019XXXXX",
"purchaseId": "a778b928b32ed0871958e8bcfb757e54f0bc894fa8df7dd8dbb553cxxxxxxxx",
"contentId": "000005059XXX",
"countryId": "USA",
"packageName": "com.abc.xyz"
},
{
"orderId": "S20230210KR019XXXXX",
"purchaseId": "90a5df78f7815623eb34f567eb0413fb0209bb04dad1367d7877edxxxxxxxx",
"contentId": "000005059XXX",
"countryId": "USA",
"packageName": "com.abc.xyz"
},
]
}
Often, the responses include all of the related details about person purchases, such because the in-app merchandise title, value, and cost standing. Subsequently, you need to use the data and create views for a better order administration.
WordIf the IAP working mode is configured to check mode, the API response is empty. It is because the API is configured to function and return a response solely in manufacturing mode.
Conclusion
You have got realized learn how to implement merchandise buy, consumption, and registration, in addition to learn how to combine the Samsung IAP Orders API and configure a server to fetch all of the cost and refund historical past inside particular dates.
Integrating the Samsung IAP Orders API performance into your server is a vital step in managing your software funds historical past to make sure a seamless expertise to customers. Now, you possibly can implement the Samsung IAP Orders API into your software to trace all funds, refunds and make your enterprise extra manageable.
For added data on this subject, see the sources beneath: